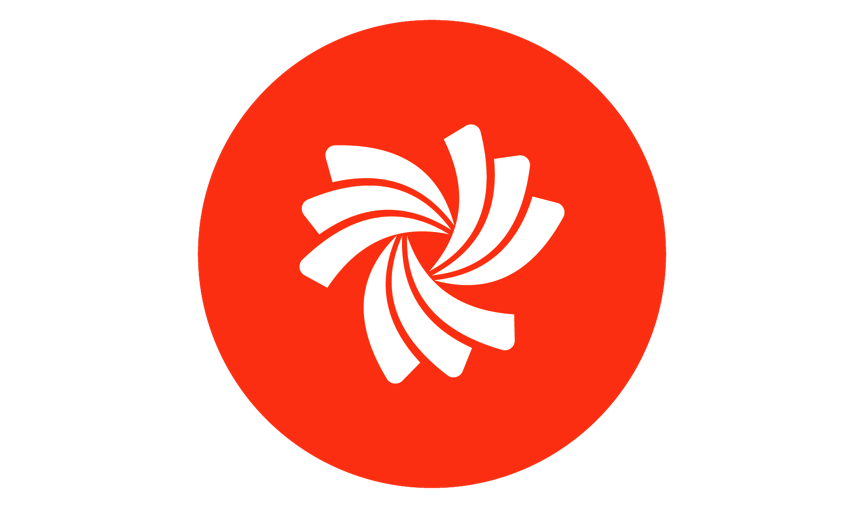
# RESTful API Workflow Examples
Below are example workflows that can be automated with the REST API.
The first two workflows, Impose and Plan, utilize the Imposition AI tools in the Jobs API. These tools can be used for estimating cost as well as generating optimized print-ready layouts. The third workflow shows how to add new stocks and sheets to the Phoenix library via the Libraries API
# Phoenix Plan Example
The quickest way to get started with the Phoenix API is to use the 'Plan' endpoint to auto-generate imposed layouts. The Plan Imposition AI tool will search across multiple press and sheet/roll combinations to find the best overall job, which might have two or more layouts.
Plan does not require a sheet to be present in the job. Instead, the Plan operation accepts a list of presses and sheets to be used in the evaulation of the most cost-effective imposed solution.
# POST Step 1 - Create New Job
For the full list of available properties, see POST Job in the API documentation.
# POST Step 2 – Add Products to Job
For the full list of available properties, see POST Product in the API documentation.
⚠️ Notice the Job 'id' 000123 from Step 1 is being used in the URL.
⚠️ If Phoenix is running on Windows OS, a backslash ("\") in the path requires an escape character ("\\"). For example:
C:\\Users\\tyler\\Desktop\\Phoenix\\jj-label.pdf
TIP
As an alternative to creating products one-by-one directly with the API, you can create several products in one shot by importing a CSV file describing each product using the CSV import API endpoint:
POST /jobs/000123/products/import/csv
{
"path": "/Users/tyler/Desktop/phoenixAPI/products-1.csv"
}
💡 See lessons for examples on the standard CSV format in Phoenix. Alternatively, you can define your own CSV format, create a corresponding preset in Phoenix, and reference this preset by name using the "preset" field.
# POST Step 3 – Run Plan
After all of the products have been successfully added to the job, run 'Plan' by specifying the presses, stocks, and defining the settings to be used for the Plan tool. Keep in mind this endpoint is asynchronous.
For the full list of available properties, see POST Plan in the API documentation.
⚠️ The Phoenix Plan endpoint will run the Plan algorithm searching legal combinations of devices and sheet sizes until either all permuations are exhausted, a maximum specified amount of time has lapsed (use the "stop-minutes" property to define the max amount of time to run plan), or a manual stop occurs by using to the '/jobs/{projectid}/plan/stop' endpoint.
# GET Step 4 – Get Plan Status
Due to the ASYNC nature of the Plan tool, you can check the current status of Plan. The status will let you know the current state of the Plan algorithm in real-time (data such as: number of results, lowest waste percentage, lowest number of layouts).
You can also see how long plan has been running as well as view errors and/or warnings.
For the full list of available properties, see GET Plan Status in the API documentation.
GET /jobs/000123/plan/status
💡 You can continue asking for the status of Plan to quickly understand the current lowest cost result, lowest waste result, and the lowest number of layouts in a result.
# GET Step 5 – Get Plan Results
Results will contain information on the quality of each plan generated by Plan as well as statistics on each layout within the plan. Optionally, you can sort, paginate, and request additional details about the Plan results such as thumbnails of the layouts, dielines, etc...
For the full list of available properties, see GET Plan Results in the API documentation.
💡 See the GET Plan Results for a full list of query parameters you can use to control the data that is returned from this call.
# POST Step 6 – Apply a Plan Result
After determining which Plan result you would like to use from the list of plan results, simply 'apply' the specific Plan result index to the job.
For the full list of available properties, see POST Apply Plan in the API documentation.
# POST Step 7 – Export Layout PDF Print File
After applying the Plan result, you can export the PDF print file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
For the full list of available properties, see POST Export PDF in the API documentation.
POST /jobs/000123/export/pdf
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_imposed.pdf"
}
⚠️ When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
# POST Step 8 – Export Layout PDF Cut File
After applying the Plan result, you can export the PDF cut/die file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
For the full list of available properties, see POST Export PDF in the API documentation.
POST /jobs/000123/export/die/pdf
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_imposed_CUT.pdf"
}
⚠️ When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
# POST Step 9 – Export JSON Report
After applying the Plan result, you can export a JSON Report file. This JSON report file provides all of the details about the imposition plan (# of sheets, costing, overruns, etc...). This report can be used by an external MIS or ERP system to eliminate production data entry.
Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
POST /jobs/000123/export/report/json
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_report.json"
}
# POST Step 10 – Export Phoenix Job File
After applying the Plan result, you can save the job (PHX) file. The Phoenix job file is useful for when additional 'manual' steps need to be performed using the Phoenix application.
For example, prepress may need to manually place a special sheet or product mark for the press.
POST /jobs/000123/save
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123.phx"
}
💡 Saving a Phoenix job file is also a good way to visualize results inside of Phoenix when debugging API workflows.
# DELETE Step 11 – Close/Delete Job
DELETE /jobs/{jobid}
DELETE /jobs/000123
⚠️ '{jobid}' is the only required url param when removing/deleting a job. In the above example, the 'jobid' = '000123'
⚠️ It is best practice to remove/delete the job after tasks are completed to alleviate unnecessary memory consumption by the Phoenix API.
# Phoenix Impose Example
The Impose algorithm is used to determine the most cost-effective Single** gang layout based on the current list of products, a specified press, and a specified sheet size. The 'Impose' Imposition AI tool differs from the 'Plan' Imposition AI tool as 'Plan' will return results containing potentially many layouts.
Using Impose is useful when you would like Phoenix to evaluate one or many products to be ran on one single press run.
# POST Step 1 - Create New job
For the full list of available properties, see POST Job in the API documentation.
# POST Step 2 – Add Products to Job
For the full list of available properties, see POST Product in the API documentation.
⚠️ Notice the Job 'id' 000123 from Step 1 is being used in the URL.
⚠️ If Phoenix is running on Windows OS, a backslash ("\") in the path requires an escape character ("\\"). For example:
C:\\Users\\tyler\\Desktop\\Phoenix\\jj-label.pdf
TIP
As an alternative to creating products one-by-one directly with the API, you can create several products in one shot by importing a CSV file describing each product using the CSV import API endpoint:
POST /jobs/000123/products/import/csv
{
"path": "/Users/tyler/Desktop/phoenixAPI/products-1.csv"
}
💡 See lessons for examples on the standard CSV format in Phoenix. Alternatively, you can define your own CSV format, create a corresponding preset in Phoenix, and reference this preset by name using the "preset" field.
# POST Step 3 – Assign Sheet to Job
For the full list of available properties, see POST Set Layout Sheet in the API documentation.
If you created an empty job or your job template did not include a sheet, you will need to assign a sheet to the job before running Impose.
POST /jobs/000123/layouts/1/sheet
{
"stock": "14pt Glossy",
"grade": "300 gsm",
"name": "36 x 28\" Long"
}
⚠️ Notice a backslash ("\") in the 'name' property is required to escape the special character (") in the name of the sheet.
# POST Step 4 – Assign Press to Job
For the full list of available properties, see POST Set Layout Press in the API documentation.
Now, you will need to assign a press to be used before running Impose.
POST /jobs/000123/layouts/1/press
{
"name": "CD 102"
}
# POST Step 5 – Run Impose
For the full list of available properties, see POST Run Impose Tool in the API documentation.
💡 Leaving a list property empty ([]) (for example, 'products') tells Phoenix to Impose using all available products in the job.
This will cause Phoenix to generate several ganged results for layout 1.
⚠️ In addition to the Imposition AI profile, you can specify a subset of products from the job to impose, as well as a subset of templates when using template-based ganging. You can also have Phoenix stop running impose after a certain number of minutes using the "stop-minutes" property.
# GET Step 6 – Get Impose Results
For the full list of available properties, see GET Impose Results in the API documentation.
Results will contain information on the quality of each imposed result generated by the Impose tool as well as statistics on each layout. Optionally, you can request thumbnails of the Impose results to display renderings of the layout with artwork, dieline, or layout with randomized colors.
💡 See the GET Impose Results for a full list of query parameters you can use to control the data that is returned from this call.
# POST Step 7 – Apply an Impose Result
For the full list of available properties, see POST Apply Impose Result in the API documentation.
To apply an Impose result to the job, do the following:
# POST Step 8 – Export Layout PDF Print File
After applying the Plan result, you can export the PDF print file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
For the full list of available properties, see POST Export PDF in the API documentation.
POST /jobs/000123/export/pdf
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_imposed.pdf"
}
⚠️ When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
# POST Step 9 – Export Layout PDF Cut File
After applying the Plan result, you can export the PDF cut/die file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
For the full list of available properties, see POST Export PDF in the API documentation.
POST /jobs/000123/export/die/pdf
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_imposed_CUT.pdf"
}
⚠️ When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
# POST Step 10 – Export JSON Report
After applying the Plan result, you can export a JSON Report file. This JSON report file provides all of the details about the imposition plan (# of sheets, costing, overruns, etc...). This report can be used by an external MIS or ERP system to eliminate production data entry.
Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
POST /jobs/000123/export/report/json
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123_report.json"
}
# POST Step 11 – Export Phoenix Job File
After applying the Plan result, you can save the job (PHX) file. The Phoenix job file is useful for when additional 'manual' steps need to be performed using the Phoenix application.
For example, prepress may need to manually place a special sheet or product mark for the press.
POST /jobs/000123/save
{
"path": "/Users/tyler/Desktop/phoenixAPI/000123.phx"
}
💡 Saving a Phoenix job file is also a good way to visualize results inside of Phoenix when debugging API workflows.
# DELETE Step 12 – Close/Delete Job
DELETE /jobs/{jobid}
DELETE /jobs/000123
⚠️ '{jobid}' is the only required url param when removing/deleting a job. In the above example, the 'jobid' = '000123'
⚠️ It is best practice to remove/delete the job after tasks are completed to alleviate unnecessary memory consumption by the Phoenix API.
# Managing Stocks Library
Stocks contain one or more grades which define thickness and/or weight. Each grade in turn contains the lists of sheets and rolls that are available.
# POST Step 1 – Create a New Stock
For this example, we will create a new stock called "Cougar Cover Cream Uncoated" and add three sheet sizes for this stock to the library.
For the full list of available properties, see POST Add Stocks in the API documentation.
POST /libraries/stocks
{
"name": "Cougar Cover Cream Uncoated",
"description": "Description of stock",
"notes": "Additional Notes",
"external-id": "A reference ID to link to external databases",
"vendor": "Cougar",
"grades": [
{
"caliper": "18pt",
"name": "130# Cover",
"weight": "130",
"weight-units": "Lb",
"weight-type": "Cover",
"sheets": [
{
"dimension1": "40in",
"dimension2": "28in",
"cost": "1670",
"cost-units": "Per1000Lb",
"grain": "Long"
},
{
"dimension1": "29in",
"dimension2": "20in",
"cost": "1660",
"cost-units": "Per1000Lb",
"grain": "Long"
},
{
"dimension1": "19in",
"dimension2": "13in",
"cost": "1660",
"cost-units": "Per1000Lb",
"grain": "Long"
}
]
}
]
}
Upon success this call returns the URL of the newly created stock which contains the auto-generated ID.
RESPONSE
{
"type": "responseEntity",
"success": true,
"status-code": 200,
"errors": [],
"warnings": [],
"resources": [
"http://localhost:8022/phoenix/libraries/v2/stocks/704a1cee-46c7-4ba7-be2f-adc19611d4ea"
]
}
# PUT Step 2 – Add a New Sheet Size
It is common for your business system (MIS or ERP System) or stock inventory management system to add a new sheet size or change the stock pricing for a certain vendor. Let's see how we can update a stock to make Phoenix aware of changes from an external system.
For this example, we will add a new sheet size (40" x 26") to the stock we created above in Step 1 called "Cougar Cover Cream Uncoated".
Below you can see we are passing the stock 'id' (704a1cee-46c7-4ba7-be2f-adc19611d4ea
) from the Step 1 response when we initially added the stock (PUT /libraries/v2/stocks/704a1cee-46c7-4ba7-be2f-adc19611d4ea
).
When updating the stock, pass the most recent information from the business system to update the stock. In our case, you can see we added a new sheet size in the
'sheets' list (40" x 26").
For the full list of available properties, see PUT Stocks in the API documentation.
PUT /libraries/v2/stocks/704a1cee-46c7-4ba7-be2f-adc19611d4ea
{
"name": "Cougar Cover Cream Uncoated",
"description": "Description of stock",
"notes": "Additional Notes",
"external-id": "A reference ID to link to external databases",
"vendor": "Cougar",
"grades": [
{
"caliper": "18pt",
"name": "130# Cover",
"weight": "130",
"weight-units": "Lb",
"weight-type": "Cover",
"sheets": [
{
"dimension1": "40in",
"dimension2": "28in",
"cost": "1670",
"cost-units": "Per1000Lb",
"grain": "Long"
},
{
"dimension1": "29in",
"dimension2": "20in",
"cost": "1660",
"cost-units": "Per1000Lb",
"grain": "Long"
},
{
"dimension1": "19in",
"dimension2": "13in",
"cost": "1660",
"cost-units": "Per1000Lb",
"grain": "Long"
},
{
"dimension1": "40in",
"dimension2": "26in",
"cost": "1660",
"cost-units": "Per1000Lb",
"grain": "Long"
}
]
}
]
}
# DELETE Step 3 – Remove a Stock from Library
⚠️ Note you use the stock ID returned from step 1 to refer to the stock and grade in the URL.
For the full list of available properties, see DELETE Stocks in the API documentation.
DELETE /libraries/v2/stocks/704a1cee-46c7-4ba7-be2f-adc19611d4ea
# Tiling Graphics Example
In this tiling example, we are asking Phoenix to auto-generate horizontal tiles that will be less than or equal to the maximum width of our roll material (60") so that the resulting print files will fit properly on the roll material.
In the example below, we will use the API to generate the horizontal tiles, while setting the vertical tiling to None
(typical use-case from our roll-based printers).
# POST Step 1 - Create new Project
Create a new Phoenix project by posting an id
to the endpoint.
You will use the ID in the upcoming calls to reference back to your project when adding products, nesting, and exporting.
POST /jobs
{
"id":"Project Name"
}
"id" is the only required property when creating a new project. Other properties can be defined as well. See API Swagger documentation (http://localhost:8022/) Method: POST
# POST Step 2 – Add a tiled product to the job
For each tiled product, create an associated product in the Phoenix project. Specify the width, height, artwork path, and tiling settings of the product. You can set different tiling setups for horizontal and vertical tiling. In our example, we are asking Phoenix to autogenerate tiles that are less than or equal to the maximum width of our roll material (60") and setting the vertical scale to None
(typical use-case from our roll-based printers)
POST /jobs/{projectid}/products
{
"name": "Tiled Product 1",
"type": "Tiled",
"ordered": "1",
"stock": "Roll",
"width": "90in",
"height": "70in",
"rotation": "Orthogonal",
"bleed-type": "Margins",
"bleed-margins": {
"bottom": ".125in",
"left": ".125in",
"right": ".125in",
"top": ".125in"
},
"min-overruns": "0",
"max-overruns": "0",
"marks": [],
"tiling": {
"type": "StandardTiling",
"start": "Top Left",
"order": "Snaking Horizontal",
"horizontal-rule": {
"type": "FixedSize",
"size": 4320
},
"horizontal-method": {
"type": "Overlap",
"overlap-rule": "Top",
"overlap": 72
}
},
"front-inks": ["C","M","Y","K"],
"back-inks": ["C","M","Y","K"]
}
HEADS-UP It's good to point out the tiles entity (tile size & tile overlap values) uses points (1 inch == 72 points).
When creating tiled products, you can specify a specific number of tiles and Phoenix will automatically compute the tile sizes.
You can also pass a customized list of tiles for Phoenix to use when generating tiles. For example, you can pass the X,Y cordinates to precisely define the tiles that Phoenix will produce.
# POST Step 3 – Run Plan
After all of the products have been successfully added to the job, run 'Plan' by specifying the presses, stocks, and defining the settings to be used for the Plan tool. Keep in mind this endpoint is asynchronous.
The Phoenix Plan endpoint will run the Plan algorithm searching all legal combinations of devices and sheet sizes until either all permuations are exhausted, a maximum specified amount of time has lapsed (use the "stop-minutes" property to define the max amount of time to run plan), or a manual stop occurs by using to the '/jobs/{projectid}/plan/stop' endpoint.
POST /jobs/{{projectId}}/plan/start
{
"presses": [
"HP Latex 3100"
],
"profiles-inline": [
{
"name": "Roll Nesting",
"strategies": {
"horizontal-cut": "true",
"vertical-cut": "false",
"die-table-cut": "false",
"free-nesting": "false",
"grid-nesting": "false",
"strip-nesting": "false",
"horizontal-strip": "false",
"vertical-strip": "false",
"templates": "false"
},
"strip-options": {
"strip-rule": "None",
"template-rule": "None",
"alignment": "TopLeft",
"gutter": "0mm",
"gutter-rule": "Always"
},
"layout-options": {
"sheet-fill": "Min",
"allow-bleed-in-gripper": "false",
"user-derived-sheets": "false",
"ordered-placement": {
"favor-ordered-placement": "true",
"start-corner": "BottomLeft",
"order-method": "HorizontalSnake"
}
},
"plan-options": {
"plan-mode": "Sequential",
"allow-product-spanning": "true"
}
}
],
"templates": [
]
}
# GET Step 4 – Get Plan status
GET /jobs/{{projectId}}/plan/status
Due to the ASYNC nature of the Plan tool, it may be required to check the current status of Plan. The status will let you know the current 'state' of the Plan algorithm in real-time. You can see how long plan has been running as well as view errors or warnings.
By creating a loop, you can continue asking for the status of Plan to quickly understand the current lowest cost result, lowest waste result, and the lowest number of layouts in a result.
# POST Step 5b. – Stop plan tool if currently running (if needed)
To manually STOP the Plan algorithm in a specific Phoenix job, simply call this endpoint to STOP Plan at its current state.
/jobs/{{projectId}}/plan/stop
# GET Step 5a. – Get list of most recent results
Method: GET
/jobs/{{projectId}}/plan/results?limit=5&start=1&sorting=Cost&layouts=true&thumb=true&plan-thumb=true&thumb-width=200&thumb-height=200&render-mode=Artwork
Results will contain information on the quality of each plan generated by Plan as well as statistics on each layout within the plan.
You can limit the results, sort results, and ask for thumbnails of the results to display in your application for the end-user.
See the Live Documentation for a full list of query parameters you can use to control the data that is returned from this call.
# Query Params
Param | value |
---|---|
limit | 5 |
start | 1 |
sorting | Cost |
layouts | true |
thumb | true |
plan-thumb | true |
thumb-width | 200 |
thumb-height | 200 |
render-mode | Artwork |
# POST Step 6 – Apply a Plan result
After determining which Plan result you would like to use, simply 'apply' the specific Plan result to the job by doing the following:
POST /jobs/{{projectId}}/plan/results/1/apply
This will apply the ###first
result ### from the Plan results list of the job. Before applying a result, use sorting params in the GET
request to determine the best result to apply.
The first result is not always the "best" result, it is important to iterate through the results to apply the result that fits your need.
To apply the third Plan result from the Plan results list, make a POST call to the endpoint
POST /jobs//plan/results/3/apply
Method: POST{{baseUrl}}/jobs/01234567/plan/results/1/apply
# GET Step 7 – Get all products of current project
GET /jobs/{{projectId}}/products?thumb=false&thumb-width=200&thumb-height=200&render-mode=Colors
After applying the Plan result, you can GET the products in the job to pull information about the product such as number of times the product is placed on the layout and the layout index (if multiple layouts are created by Plan) for which the product is imposed on.
See the Live Documentation for a full list of query parameters you can use to control the data that is returned from this call.
# Query Params
Param | value |
---|---|
thumb | false |
thumb-width | 200 |
thumb-height | 200 |
render-mode | Colors |
# POST Step 8 – Export the layout PDF print file
After applying the Plan result, you can export the PDF print file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
POST phoenix/jobs/{{projectId}}/export/pdf
{
"path": "D:\\Users\\tyler\\Desktop\\PhoenixDebug\\{{projectId}}_PRINT.pdf"
}
When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
# POST Step 9 – Export the layout PDF Cut file
After applying the Plan result, you can export the PDF cut file. Keep in mind the 'path' property is the full unc path which needs to be accessible by the Phoenix computer.
POST phoenix/jobs/{{projectId}}/export/die/pdf
{
"path": "D:\\Users\\tyler\\Desktop\\PhoenixDebug\\{{projectId}}_CUT.pdf"
}
When using a backslash ("\") in the path, don't forget to escape the backslash character with a backslash ("\\"). For example:
C:\\Users\\tyler\\Desktop\\PhoenixOutput\\imposed.pdf
Method: POST
# POST Step 10 – Export Phoenix Project File
From here you can save the project (PHX) file.
POST /jobs/{{projectId}}/save
{
"path": "D:\\Users\\tyler\\Desktop\\PhoenixDebug\\{{projectId}}.phx"
}
# POST Step 11 – Export Tiling Installation Report
Phoenix will auto-generate a tiling report PDF for installation purposes. This can be used for installers to know the order/sequence of the tiles that have been shipped.
POST /jobs/{{projectId}}/save
{
"path": "D:\\Users\\tyler\\Desktop\\PhoenixDebug\\{{projectId}}_InstallReport.pdf"
}
# DELETE Step 12 – Close the Phoenix job
DELETE /jobs/{{projectId}}
"{projectId}" is the only required url param when removing/deleting a job.
It is best practice to remove/delete the job after tasks are completed to alleviate unnecessary memory consumption by the Phoenix API.