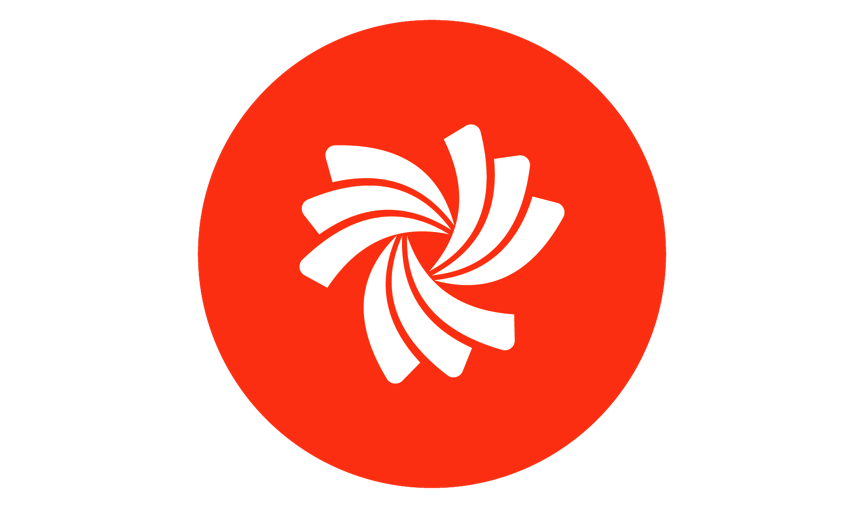
# Hotfolder Scripting
Hot folders automation includes an optional scripting interface, providing an unprecedented amount of customization during automation. Scripting is done in the popular JavaScript language.
There are several uses for scripting in hot folders. Below are some of the more common examples:
- Support input files with formats other than the standard Resources XML format described in this document. For example, another XML format, CSV spreadsheet, or other text-based format containing information about the job to be automated.
- Waiting for a certain number of input files to come into the hot folder or a certain duration before performing actions.
- Analyzing the results of ganging tools to remove layouts that do not meet certain criteria such as sheet usage or waste and moving products from these layouts back into the input file to be ganged with more incoming files.
- Customized notification when a job has succeeded or failed. Provided utility methods in the scripting environment make it easy to send email alerts or record error details in local files.
- Convert image files to PDFs or breaking up large PDFs into tiles using the PDFUtils utility methods.
# Scripting Hooks
Hot folder scripting has three entry points that you can implement to add custom behavior to hot folders: onStart(), onSuccess(), and onFail(). All three functions are optional, but at least one of these functions needs to be implemented in a script when it is loaded into a hot folder.
# onStart()
Description: Initial hook when a file comes into the hot folder. This function is called before any actions are processed to convert the incoming file into a list of resource objects needed by the actions in the hot folder, perform tiling or image to PDF conversion, delay processing based on a custom trigger event, and more. Trigger Event: Called when a file comes into the hot folder with file extension matching the file-type attribute in <phoenix-actions> element, or .xml if file-type is not specified. Arguments:
- state (FolderState): Hot folder state
- inputs (InputFile List): List of InputFiles
- Return Type: A JavaScript array of resource objects needed by actions. These are the same resource objects that are normally represented in Resources XML files. Note: As mentioned in the Actions XML Format section, by default hot folders watch for files with the .xml extension. If the file format you are processing in onStart() does not have a .xml extension you need to specify the expected extension in the Actions XML file in the <phoenix-actions> element attribute called "file-type". For example, if the incoming file in the hot folder is a CSV file with extension .csv, the Actions XML needs to specify the csv extension:
<phoenix-actions version="1.0" file-type="csv">
# onSuccess()
Description: Hook called when all actions in the hot folder have completed successfully. This hook is useful for sending notification emails, adding custom logging, moving per-layout export files into folders organized by presses/stocks, moving products in sub-par layouts back into the input folder, and more. Trigger Event: All actions in the hot folder have completed successfully for a given task. Arguments:
- state (FolderState) – Hot folder state (see section 3.5.2)
- inputs (InputFile List) -List of InputFiles (see section 3.5.3)
- job (JobEntity) – Job object representing detailed information about the job that completed. Job entity format is the same as the JSON job report. You can find the formal schema definition in this guide under the Schemas folder. Return Type: None
# onFail()
Description: Hook called when an error occurred during hot folder processing. This hook is useful for sending notification emails or recording the event by other means. Trigger Event: An error occurred during processing for a given task. Arguments:
- state (FolderState) – Hot folder state (see section 6.2)
- inputs (InputFile List) -List of InputFiles (see section 6.3)
- report (ErrorReport) – Error report listing errors and warnings that occurred during task processing. Error Report format formal schema definition in this guide under the Schemas folder. Return Type: None
# Classes
# FolderState Class
Method | Description | Arguments | Return Type |
---|---|---|---|
getName() | Returns the HotFolder name | None | Hotfolder name as a String |
getTaskId () | Method for getting the task ID. Task IDs are automatically generated unique IDs used for tracking and logging. | None | Task Id as a string |
getOutputFolder() | Method for getting the output folder | None | Configured output folder path as a string |
getData() | Method for retrieving values from the data store for this hot folder. Data store can be used in scripting to record state across scripting hook calls | key (String) – the key for the desired value | Data value as a string |
setData() | Method for recording values in the data store | key (String) – the key to associate with the value value (String) - value being added to the map | None |
removeData() | Method for removing values from the data store | key (String) – the key for the value being removed | None |
debug() | Method for adding a debug message to this hot folder's logs. Log messages will appear in Hot Folder View. | message (String) – message to be added | None |
info() | Method for adding a info message to this hot folder's logs | message (String) – message to be added | None |
error() | Method for adding a error message to this hot folder's logs | message (String) – message to be added | None |
# InputFile Class
Input file class representing a single input file in a hot folder
Method | Description | Arguments | Return Type |
---|---|---|---|
isProcessed() | Method returning whether the file was processed | None | Boolean. Returns true if the file has been processed |
getName() | Method gets file name | None | File name as a String |
getPath() | Method gets file path | None | File path as a String |
getLastModified() | Method gets time stamp of when the file arrived in the input folder | None | Long (Time Stamp) |
moveToProcessed() | Method to move an input file to the processed state and corresponding processed folder | None | Boolean, returns true if file is successfully moved |
moveToErrors() | Method to move file to Errors Folder | None | Boolean, returns true if file is successfully moved |
moveToInput() | Method to move file to Input Folder | None | Boolean, returns true if file is successfully moved |
# Mail Class
Method | Description | Arguments | Return Type |
---|---|---|---|
getHostname() | Gets the Hostname | None | Hostname as a string |
setHostname() | Sets the Hostname | hostname (String) | None |
getSmtpPort() | Gets the SMTP port number | None | SMTP port number as a string |
setSmtpPort() | Sets the SMTP port number | number (Int) – SMTP port number | None |
setAuthentication() | Sets the username and password for authentication | username (String) password (String) | None |
setFrom() | Sets the from address | address (String) – From address | None |
setSubject() | Sets the email subject | subject (String) | None |
setMessage() | Sets the email message text | message (String) | None |
addTo() | Adds a recipient address | address (String) | None |
addCc() | Adds a Cc address | address (String) | None |
addBcc() | Adds a Bcc address | address (String) | None |
send() | Sends the email | None | None |
setUseTls() | Sets the use of Transport Layer Security (TLS) | useTls (Boolean) | None |
setUseSsl() | Sets the use of Secure Sockets Layer (SSL) | useSsl (Boolean) | None |
getSslSmtpPort() | Gets the SSL SMTP port number | None | SSL SMTP port number as a string |
setSslSmtpPort() | Sets the SSL SMTP port number | Number (Int) – SSL SMTP port number | None |
addHeader() | Adds a header to the email | name (String) – Header name value (String) – Header value | None |
# Document Class
Document class representing a PDF document
Method | Description | Arguments | Return Type |
---|---|---|---|
pageCount() | The page count of the document | None | Number – The number of pages in the document |
page() | Page in the document | pageNumber (Number) – page number to return | Page class – Page for the page number specified |
pages() | Pages in the document | None | Array of pages |
save(String path) | Save the document | Path (String) – Path to save document to | None |
close() | Close the document | None | None |
# Page Class
Page class representing a page of PDF document
Method | Description | Arguments | Return Type |
---|---|---|---|
getMediaBox() | Gets the mediaBox Rect of the PDF page | None | Rect |
setMediaBox() | Sets the mediaBox Rect on the PDF page | mediaBox (Rect) | None |
getCropBox() | Gets the cropBox Rect of the PDF page | None | Rect |
setCropBox() | Sets the cropBox Rect on the PDF page | cropBox (Rect) | None |
getTrimBox() | Gets the trimBox Rect of the PDF page | None | Rect |
setTrimBox() | Sets the trimBox Rect on the PDF page | trimBox (Rect) | None |
getBleedBox() | Gets the bleedBox Rect of the PDF page | None | Rect |
setBleedBox() | Sets the bleedBox Rect on the PDF page | bleedBox (Rect) | None |
getArtBox() | Gets the artBox Rect of the PDF page | None | Rect |
setArtBox() | Sets the artBox Rect on the PDF page | artBox (Rect) | None |
# Rect Class
Input file class representing a single input file in a hot folder
Method | Description | Arguments | Return Type |
---|---|---|---|
getX() | Returns the x value of the Rect position | None | Double |
setX() | Sets the x value of the Rect position | x (Double) | None |
getY() | Returns the y value of the Rect position | None | Double |
setY() | Sets the y value of the Rect position | y (Double) | None |
getWidth() | Returns the width of the Rect | None | Double |
setWidth() | Sets the width of the Rect | width (Double) | None |
getHeight() | Returns the height of the Rect | None | Double |
setHeight() | Sets the height of the Rect | height (Double) | None |
# Utility Classes
Several utility classes are provided within the scripting environment for moving and parsing files, working work PDFs, sending email notifications and more.
# JobUtils
Method | Description | Arguments | Return Type |
---|---|---|---|
open() | Opens a Phoenix job (PHX) at the given path and returns the full Job entity for that job | path (String) – File path | Job Entity object representing opened job or thrown exception on failure |
artworkFiles() | Opens a Phoenix job (PHX) at the given path and returns all original artwork file paths referenced in the job | path (String) – File path | Array (String) of artwork files path |
# FileUtils
Method | Description | Arguments | Return Type |
---|---|---|---|
tempPath() | Returns the standard system temporary directory | File directory as a string | |
tempFile() | Creates a temporary file and returns the full path | File path as a string | |
mkDir() | Create a new local directory with the given path | Path (String) File path | Boolean returns true if directory was successfully created |
filename() | Retrieves the file name | path (String) – File path | File name as string excluding parent folders |
basename() | Retrieves the base file name | path (String) – File path | Base file name as string |
directory() | Retrieves the file's directory | path (String) – File path | File directory as a string excluding file name |
isDirectory() | Check if path is a directory | path (String) – File Path | Boolean returns true if path is a directory |
isFile() | Check if path is a file | path (String) – File Path | Boolean returns true if path is a File |
copyFile() | Copies file from 'source' to 'destination' | source (String) – The source file destination (String) – The destination of the file being copied | Boolean, returns true on successful copy |
moveFile() | Moves 'source' file to 'destination' | source (String) – The source file destination (String) – The destination of the file | Boolean, returns true on successful move |
concat() | Appends the file name to the base path | base path (String) filename (String) | Returns combined base path and file name as a string |
text() | Extracts all text from a file into a single string | path (String) – File path encoding (String) – Optional text encoding, defaults to UTF-8 if omitted | file text as a string |
lines() | Extracts all text from a file into a list of strings for each line in the file | path (String) – File path encoding (String) – Optional text encoding, defaults to UTF-8 if omitted | File text as a list of strings |
files() | Lists all files of given types in the specified directory | directory (String) – Directory to search in extensions (String) – Optional extension of file types to include, defaults to all file types recurse (Boolean) – Optional search for files recursively in sub-folders | List of file paths |
csv() | Generates a list of string arrays from a CSV file's values with each array representing a row of the CSV file | path (String) – Path of CSV file | CSV values as a list of arrays of string cell values |
xml() | Reads an XML file into a DOM object | path (String) – Path of XML file | DOM Object |
find() | Retrieves file with 'filename' in given 'directory' searching recursively into child directories | directory (String) – File directory filename (String) – File name | Absolute path of the file as a string |
delete() | Deletes a file | path (String) – Path of file to be deleted | None |
# MailUtils
Method | Description | Arguments | Return Type |
---|---|---|---|
create() | Creates a new email | hostname (String) – Server host name port (Int) – Port number (optional), defaults to 25 | Mail Object |
# PDFUtils
Method | Description | Arguments | Return Type |
---|---|---|---|
Open() | Opens a PDF document | path (String) – Path to the PDF document | Document |
createFromImage() | Create PDF document from an image | path (String) – Image file path | Document |
createFromImage() | Create PDF document from an image | path (String) – Image file path width (String) – Document width in points height (String) - Document height in points | Document |
createTiles() | Create tiled PDF document | path (String) – Path of Document to be tiled rects (Rects List) – Tile rects bleed (String) – Tile bleed | Document |
createTiles() | Create tiled PDF document | document (String) –Document to be tiled rects (Rects List) – Tile rects bleed (String) – Tile bleed | Document |
impose() | Impose a multi-page artwork file using a given folding pattern and binding method, saving the resulting PDF file to a given output path with option to separate cover | artwork (String) – Input artwork file foldingPattern (String) – Name of folding pattern in Folding library to use while imposing artwork pages bindingMethod (String) – Name of binding method being used pageMargins (Margins) – Sheet margins to add faceTrim (String) – Amount of face trim headTrim (String) – Amount of head trim footTrim (String) – Amount of foot trim spineSize (String) – Size of book spine separateCover (String) – If set to "true" exports the cover pages of the artwork to a separate file with "-cover" postfix added to output path output (String) – Output path to save imposed PDF to | None |
saveSubSet() | Opens a PDF file at inputPath and saves all the pages between startPage and endPart inclusively to an outputPath as a new PDF | path (String)- Path of Document Name (String) Output name Start(Int) start page End(Int) end page | Document |
# GeomUtils
Method | Description | Arguments | Return Type |
---|---|---|---|
rect() | Creates new Rect | None | Rect |
rect() | Creates a Rect at position (x,y) of dimensions ('width' X 'height') | x (Double) – x value of the Rect y (Double) – y value of the Rect width (Double) – Rect width height (Double) – Rect height | Rect |
# Example Hotfolder Scripts
Below you'll find some example scripts and snippets to help you understand how scripting works, as well as help with some common scripting needs
# Find a product's custom property
You can access a product's default properties fairly simply, by using product.index
or product.name
. If you want to access a custom property, however, they are stored in a properties list inside of the product. An easy way to find your property is to loop through the custom properties and return the value if the property name matches the one you're looking for:
// Grab first product to find product properties
// required for file naming. Could place this in
// a loop to look through all products
var product = job.products.get(0);
// Loop through all of this product's properties,
// saving StoreNum property values
for (var i=0; i<product.properties.size(); i++) {
var property = product.properties.get(i);
if (property.name == "StoreNum"){
var store = property.value;
}
}